Advanced Randomization
Setup script to randomize all questions
#set($ary = ["Q3", "Q4", "Q5", "Q6","Q7","Q8"]) #set($ary = $survey.randomizeList($ary)) #foreach( $val in $ary) $survey.branchTo("$val") #endIn the above script in the first statement questions Q3, Q4, Q5, Q6, Q7 and Q8 are added to a list and randomized in the second statement. Once randomized the survey branches to each question randomly. Steps for setting this up:
- Setup the basic survey with all the Questions.
- Now to randomize questions 3 through 8 add the Custom Scoring/Logic question just before Q3
- Enter the above script in the Custom Scoring / Logic question.
- For Randomization to work there has to be a common block termination question with which the Survey should continue. In the above instance the Block Termination Question is Q9
- Branch each of the Question in the above list i.e. Q3, Q4, Q5, Q6, Q7 and Q8 to Q9 which is the Block Termination Question and test the Survey!
Setup Randomization to randomly select 3 questions from a pool of 6 questions (N out of M)
#set($ary = ["Q3", "Q4", "Q5", "Q6","Q7","Q8"]) #set($ary = $survey.randomizeList($ary, 3)) #foreach( $val in $ary) $survey.branchTo("$val") #endIn the above script questions Q3, Q4, Q5, Q6, Q7 and Q8 are added to a list and randomized. After randomization only 3 questions are returned to the list randomly and subsequently the survey branches to only those 3 questions. Steps for setting this up:
- Setup the basic survey with all the Questions.
- Now to randomize questions 3 through 8 add the Custom Scoring/Logic question just before Q3
- Enter the above script in the Custom Scoring / Logic question.
- For Randomization to work there has to be a common block termination question with which the Survey should continue. In the above instance the Block Termination Question is Q9
- Branch each of the Question in the above list i.e. Q3, Q4, Q5, Q6, Q7 and Q8 to Q9 which is the Block Termination Question and test the Survey!
You can use the same logic for Randomizing entire Blocks of Questions. To do so add only the first question in each block for Randomization and branch the last question of each block to the common block termination question.
Piping text
How to Pipe Text over multiple pages?
Example 1: Q1 Which sport do you play the most? Baseball Rugby Soccer Basketball Other __________In the above example, you wish to ask respondents subsequent questions based on the sport they play. The in-built piping option will only work on the next immediate page. Also you cannot automatically pipe the text entered if other option is choosen. To set up the above Survey you will need to use Custom Scoring/Logic Script type question. For this you will need to update the Custom Variable 1 with the Sport and you can then base the branching on the selection and also use the text anywhere in the Survey.
The script for the above requirement is as follows:
#if (${Q1} == 1) $survey.updateCustom1("Baseball") $survey.branchTo("Q2") #end #if (${Q1} == 2) $survey.updateCustom1("Rugby") $survey.branchTo("Q2") #end #if (${Q1} == 3) $survey.updateCustom1("Soccer") $survey.branchTo("Q2") #end #if (${Q1} == 4) $survey.updateCustom1("Basketball") $survey.branchTo("Q2") #end #if (${Q1} == 5) $survey.updateCustom1("${Q1_OTHER}") $survey.branchTo("Q2") #endIn the above script we check which option is selected for Q1 and depending on that we update the Custom1 variable with the correct text. If option 5 that is the Other option is selected then the Custom1 variable will get updated with the user input text. To reference the text entered for the Other option we use: "QuestionCode_OTHER" so in the above example QuestionCode is Q1 so we reference the User entered text using Q1_OTHER. We are also branching to the next question which is Q2 for each selection. If required we can branch to different questions based on the selection. You can use ${custom1} to replace the value stored in Custom1 variable. Once updated the Custom1 value can be used anywhere on the survey.
Following are the steps for setting this up:
- Setup the basic survey with all the questions
- Wherever you wish to replace the text for the sport which the user/respondent selected use ${custom1}.
- After Q1 add a Custom Scoring/Logic Script type question and enter the above script.
- Make sure there is a page break on Q1
Delayed Branching
Delayed Branching Senario:
When you use the Branch option under the tools console the Branching is executed immediately. The above example is that of delayed branching.
The script for the above requirement is as follows:
#if(${Q2} == 1) $survey.branchTo("Q10") #end #if(${Q2} == 2) $survey.branchTo("Q11") #endIn the above script: Q2, Q10 and Q11 are question codes for the respective questions. The if statement checks if answer to Q1 was 1 (Male) or 2 (Female) and accordingly branches to Q10 or Q11
Following are the steps for setting this up:
- Set up the basic survey with all the questions
- Add the Custom Scripting/Scoring logic question after Q9 in the Survey
- Set up the above Script in the Logic Question
- Set up default Branching for Q10 and Q11 to Q12
- Make sure there is a page break on Q9
How to check if a certain question is not answered?
#if(!${Q1}) $survey.branchTo("Q3") #else $survey.branchTo("Q2") #endThe above script checks if Q1 is answered or not. If Q1 is not answered then the survey branches to Q3, else it branches to Q2.
Scoring
What is Scoring Logic?
How to set up Scoring?
Consider the following example:
Q1: What is the Capital of the US?- New York
- Seattle
- Washington DC
- Chicago
- 20
- 45
- 50
- 63
- 4th, June
- 4th, July
- 14th, July
- 24th, Jan
- Washington, George
- Adams, John
- Jefferson, Thomas
- Madison, James
- Canada
- France
- England
- Russia
- Q1 = 3
- Q2 = 3
- Q3 = 2
- Q4 = 1
- Q5 = 2
The Script for Scoring will be as follows:
#set($tot=0) #if (${Q1} == 3) #set($tot = $tot + 1) #end #if (${Q2} == 3) #set($tot = $tot + 1) #end #if (${Q3} == 2) #set($tot = $tot + 1) #end #if (${Q4} == 1) #set($tot = $tot + 1) #end #if (${Q5} == 2) #set($tot = $tot + 1) #end #set($score = $tot)
How to calculate score for a Matrix Question?
#set($score=${Q1_1} + ${Q1_2} + ${Q1_3}) [This will calculate the score for the Matrix Q1] #set($score=${Q2_1} + ${Q2_2} + ${Q2_3}) [This will calculate the score for the Matrix Q2] Score can be referenced directly using the Question Code. Score for Matrix Q1: $Q1 Score for Matrix Q2: $Q2 The above example uses default values for the score. You can also set up custom score values for each individual option.
Can I display the computed score to the end-user?
- Login » Surveys » (Select Survey) » Edit Survey » Settings » Finish Options
Can I (as the administrator) view the computed score for an individual?
- Login » Surveys » Analytics » Data Management » Response Viewer
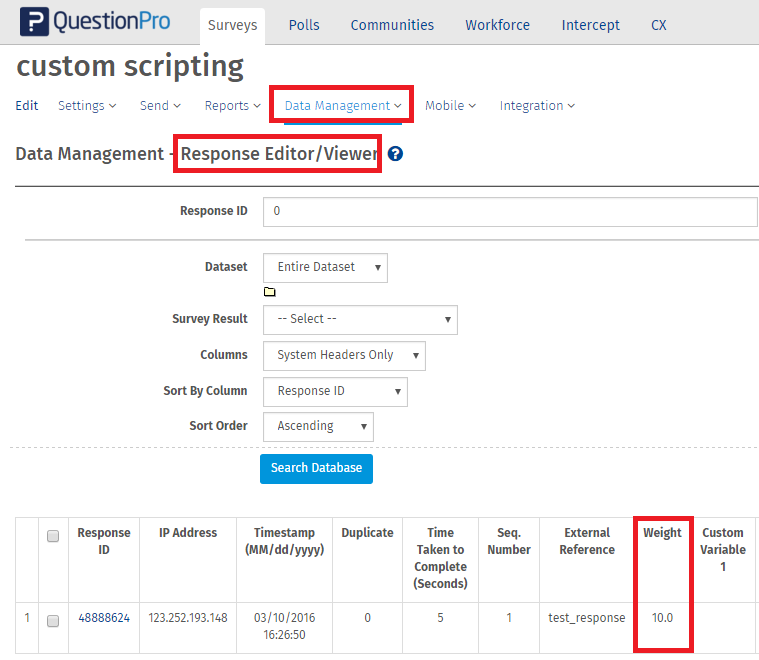
Branch Open Ended Questions
Example 1: You ask respondents which Restaurant they like to go for dinner and subsequently you wish to ask them questions related to the Restaurant of their choice.
$survey.updateCustom1("${Q1_1}") $survey.branchTo("Q2")Here Q1 is the Question Code for the Open Ended / Text input type question. And we reference the value by Q1_1 which is the first text box/field for the Question (There can be multiple text boxes for a single question. Q2 is the next question in sequence. When respondents enter the name of the Restaurant it is saved in Q1_1. Custom Variable 1: Custom1 is updated with this value and now can be used anywhere in the rest of the Survey.
Directions for setting up the Survey:
- Setup the basic survey with all the questions
- In the first question Q1 ask the respondents to entere the Restaurant of their choice. In the rest of the survey, wherever you wish to use the text entered use ${custom1} and enable Dynamic Replacement for the questions.
- After Q1 add a Custom Scoring/Logic Script type question and enter the above script.
- Make sure there is a page break on Q1
Example 2:
The Script is as follows:
#if (${Q2_1} == "0") $survey.branchTo("NewBlock") #end #if (${Q1_1} != "0") $survey.branchTo("Q3") #endThe above Script checks if the user input is 0. If the user enters 0 they are branched to the next questions starting with QuestionCode NewBlock. If the user entered value is not 0 then they are branched to Q3 and asked followup questions.
Branch Multiple Select Questions
Consider the following scenario:
Q1: Which games do you play? Baseball Basketball Soccer Tennis
The above question is a Multiple Select type question. Now, if respondents select the answer option Baseball and Basketball you want to branch them to Q2, else you want them to branch to Q3.
The script for the above example is as follows:
#if (${Q1_1} == "1" ) #if (${Q1_2} == "1") $survey.branchTo("Q2") #else $survey.branchTo("Q3") #end #end
What is the difference between referencing a multiple select type question (Check Box) versus single select question (Radio Button)?
If the question is a Multiple Select type question then the answer options are referenced as follows:
- First answer option: ${Q1_1} == "1", Second answer option: ${Q1_2} == "1", Third answer option: ${Q1_3} == "1" and so on...
- To check if the answer option was selected, check if it equals to "1" for example: #if (${Q1_1} == "1"). Unlike single select question type 1 should be within quotes.
If the question is a single select type question then the answer options are referenced as follows:
- First answer option: #if (${Q1} == 1), Second answer option: #if (${Q1} == 2), Third answer option: #if (${Q1} == 3) and so on...
- To check if the answer option is selected, check it with the position of the answer option. Quotes are not necessary in this case.
Branch Matrix Type Questions
Consider the following Matrix question:
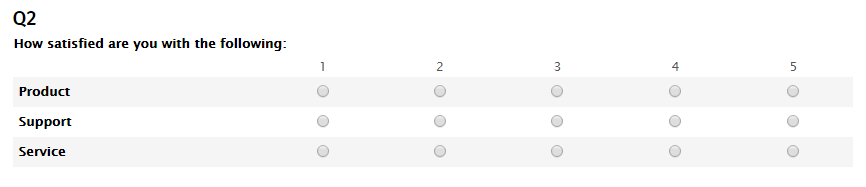
Scenario: You ask respondents to rate there satisfaction level for Product, Support and Service. If respondents give a low rating of 1 or 2 then they should be asked to comment why they gave a low rating.
The script for the above requirement is as follows:
#if (${Q2_1} == 1 ) $survey.branchTo("Q3") #end #if (${Q2_1} == 2) $survey.branchTo("Q3") #end #if (${Q2_2} == 1 ) $survey.branchTo("Q4") #end #if (${Q2_2} == 2) $survey.branchTo("Q4") #end #if (${Q2_3} == 1 ) $survey.branchTo("Q5") #end #if (${Q2_3} == 2) $survey.branchTo("Q5") #endHere: Q2 is the question code for the Matrix Question. The questions in a matrix are referenced in the following way: Q2_1 for the first question in the matrix. Q2_2 for the second and so on. The first statement checks if Q2_1 is scored as 1 or 2, if true then branching is set to Q3. Similarly the second and third if statement checks if the second question and the third question were scored as 1 or 2 and branch to the corresponding questions if true.
Following are the steps for setting this up:
- Set up the basic survey with all the questions
- Add the Custom Scripting/Scoring logic question after Q2 in the Survey
- Set up the above Script in the Logic Question
- Using the built in branching option set up default Branching for Q3, Q4 and Q5 to Q6. Here Q6 is the question code for the question with which the Survey should continue with.
- Make sure there is a page break on Q2